LlamaGym is an innovative tool that simplifies the process of fine-tuning large language model (LLM) agents through reinforcement learning (RL). Just as OpenAI’s Gym was created to standardize and simplify RL environments, LlamaGym helps make LLM agents easily usable in RL environments. In this article, we will delve into the features and usage of LlamaGym.
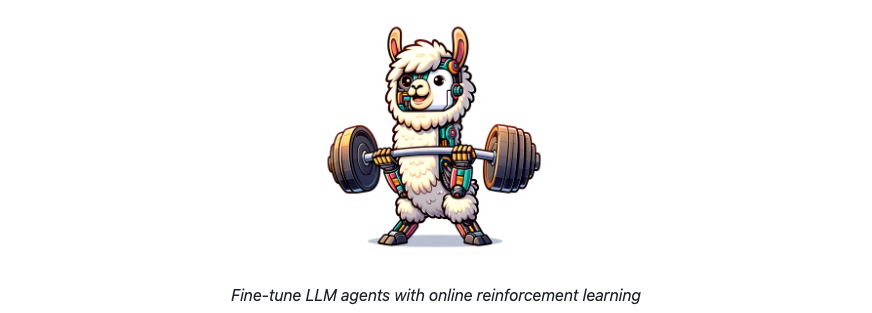
Key Features and Benefits of LlamaGym
1. Agent Abstract Class
The core of LlamaGym is a single agent abstract class. This allows users to quickly experiment with and iterate on agent prompts and hyperparameters. This abstract class includes three key methods: system prompt, observation formatting, and action extraction.
Example Implementation of BlackjackAgent Class
from llamagym import Agent
class BlackjackAgent(Agent):
def get_system_prompt(self) -> str:
return "You are an expert blackjack player."
def format_observation(self, observation) -> str:
return f"Your current total is {observation[0]}"
def extract_action(self, response: str):
return 0 if "stay" in response else 1
This class is tailored for a blackjack game. Users can define the agent’s role through the system prompt, convert the game state to text via observation formatting, and analyze the model’s response to extract the appropriate action.
2. Easy Model and Tokenizer Setup
LlamaGym simplifies the process of setting up the base LLM and instantiating the agent. Users can easily load pre-trained models and tokenizers and generate agents based on them.
Example Setup for Llama-2-7b Model
model = AutoModelForCausalLMWithValueHead.from_pretrained("Llama-2-7b").to(device)
tokenizer = AutoTokenizer.from_pretrained("Llama-2-7b")
agent = BlackjackAgent(model, tokenizer, device)
This process is similar to traditional LLM fine-tuning tasks. Users load the Llama-2-7b model and its tokenizer, then pass them to the agent class to create the agent. This allows users to easily run agents in an RL environment.
3. Reinforcement Learning Loop
LlamaGym simplifies the process of writing reinforcement learning loops. This loop operates by having the agent interact with the environment, receive rewards, learn, and perform training at the end of episodes.
Example RL Loop in Blackjack Environment
env = gym.make("Blackjack-v1")
for episode in trange(5000):
observation, info = env.reset()
done = False
while not done:
action = agent.act(observation) # Act based on observation
observation, reward, terminated, truncated, info = env.step(action)
agent.assign_reward(reward) # Assign reward to agent
done = terminated or truncated
train_stats = agent.terminate_episode() # Perform training at end of episode
This code demonstrates the process of an agent interacting with the environment and learning from rewards. The agent selects actions based on observations and learns from the rewards provided by the environment. Through this process, users can continually improve the agent’s performance.
Cautions When Using LlamaGym
- Hyperparameter Tuning: Convergence in reinforcement learning can be very difficult, so hyperparameters may need adjustment.
- Supervised Learning: Performing a supervised learning phase on sampled trajectories before running RL can improve model performance.
- Simplicity vs. Efficiency: LlamaGym prioritizes simplicity, so it may be less efficient computationally compared to other tools.
1. Importance of Hyperparameter Tuning
In reinforcement learning, hyperparameters play a crucial role. They directly affect the learning speed and performance of the agent. Therefore, users must experiment to find the optimal hyperparameters. LlamaGym facilitates these experiments for users.
2. Combining Supervised and Reinforcement Learning
Performing supervised learning on sampled trajectories before starting reinforcement learning can help the agent learn more quickly and effectively. This reduces instability in the RL process and improves initial performance.
3. Balancing Simplicity and Efficiency
LlamaGym emphasizes simplicity to enhance user accessibility. However, this may result in lower computational efficiency compared to other advanced tools. Users should select appropriate tools based on their needs.
Conclusion
LlamaGym is an innovative tool that combines reinforcement learning and LLM agents, allowing users to fine-tune and experiment with agents more easily. This enables users to maximize the potential of LLMs in RL environments. Reinforcement learning is a powerful method for significantly improving LLM performance, and LlamaGym provides an excellent starting point for this purpose.