Game development requires effective state management as a crucial element, especially when creating 3D games with Three.js. Managing various states like character health, abilities, and game progress is essential to ensure the game’s quality.
Three.js and State Management
Three.js is a powerful library that enables 3D graphics in web browsers using JavaScript.
State Management in React
Have you used React? If so, you understand the importance of state management. Managing the state of each component efficiently to update the screen appropriately whenever a specific state changes is crucial in React. To do this, React uses tools called hooks. By utilizing hooks like useEffect, you can create your own custom hooks. Custom hooks are highly reusable and can even be turned into libraries.
What is R3F?
R3F, or React Three Fiber, is a powerful library that enables the use of Three.js for 3D graphics within React. It abstracts the complexity of Three.js, making it easier for developers familiar with the React ecosystem to handle 3D graphics.
R3F is a library that renders 3D graphics in web applications using Three.js based on React, making the powerful JavaScript library Three.js more accessible for creating 3D content on the web.
Key Features
- Leveraging React’s Advantages:@b] You can use React’s state management and component-based architecture, allowing you to write structured and reusable code for complex 3D scenes.
- Component-Based Development: With R3F, you can define 3D objects, lights, and cameras as React components, enabling you to assemble 3D scenes in a React manner.
- Declarative Approach: Unlike Three.js’s imperative approach, R3F takes a declarative approach, making the code more intuitive.
Advantages
- Increased Productivity: Developers already familiar with React can quickly adapt as they use the React ecosystem.
- Reusability: The component-based architecture enhances code reusability.
- Flexibility: While using all the features of Three.js, you can write more concise and understandable code.
Example Code
Here is an example of rendering a simple 3D cube using R3F:
import React from 'react';
import { Canvas } from '@react-three/fiber';
import { Box } from '@react-three/drei';
function App() {
return (
<Canvas>
<ambientLight />
<pointLight position={[10, 10, 10]} />
<Box position={[-1.2, 0, 0]} />
<Box position={[1.2, 0, 0]} />
</Canvas>
);
}
export default App;
This code renders two 3D cubes, where the `Canvas` component contains the R3F 3D scene, and the `Box` component, provided by the Drei library, creates a 3D box.
React Three Fiber is a highly useful tool for developers looking to integrate 3D graphics into React applications, combining the strengths of React and the powerful features of Three.js to build complex 3D scenes more easily.
Why is Global State Management Necessary?
For instance, consider an error popup. The error state originates from the upper program and is passed to the popup. However, when all components need to share this state, manually managing inheritance relationships is cumbersome. Redux solves this by being a centralized state management tool, allowing all components to access and change the state. Combining redux with R3F enables efficient state management.
zustand: Global State Management for R3F
zustand is a simpler and faster state management library than redux. Its concise interface is useful when combined with R3F. Although the user communities for R3F and zustand are still growing, utilizing these two tools can lead to more efficient state management. The main page of zustand features a peaceful image of a bear playing a guitar, representing its simplicity and efficiency.
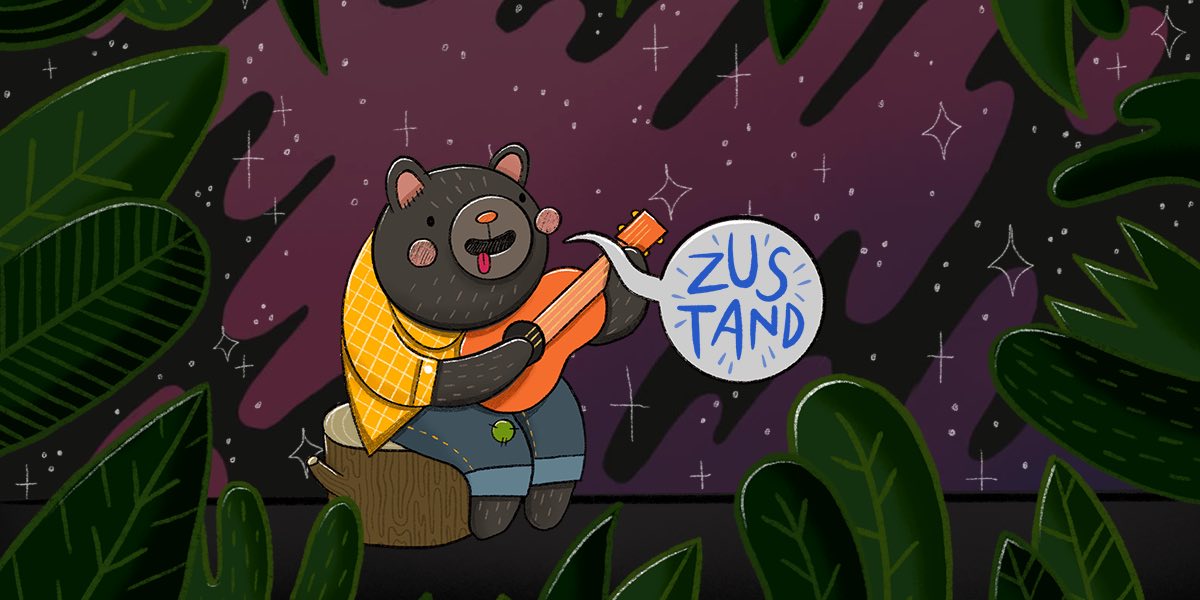
State Management in Games
State management is essential in games. For example, in RPG games, character health and abilities need to be shared throughout the game. If health reaches zero, the game ends, and whether you can use an item depends on comparing it with your abilities. Implementing these patterns requires systematic state management.
Even in a simple ball-rolling game, state management plays a crucial role. The game states can be defined as ‘ready,’ ‘in progress,’ and ‘end.’ If you consider moving the ball to the starting line in the ‘ready’ state, progressing the game in the ‘in progress’ state, and ending the game in the ‘end’ state, using zustand to manage these states can efficiently control the game’s flow.
Conclusion
As seen above, game development with Three.js offers many possibilities. Efficient state management leads to more enjoyable and stable games. Why not create your own amazing game using Three.js and zustand right now?