Web applications rely heavily on real-time communication to enhance user experience. WebSocket is a powerful technology that enables effective real-time communication. In this article, we will delve into the basic concepts and implementation methods of WebSocket.
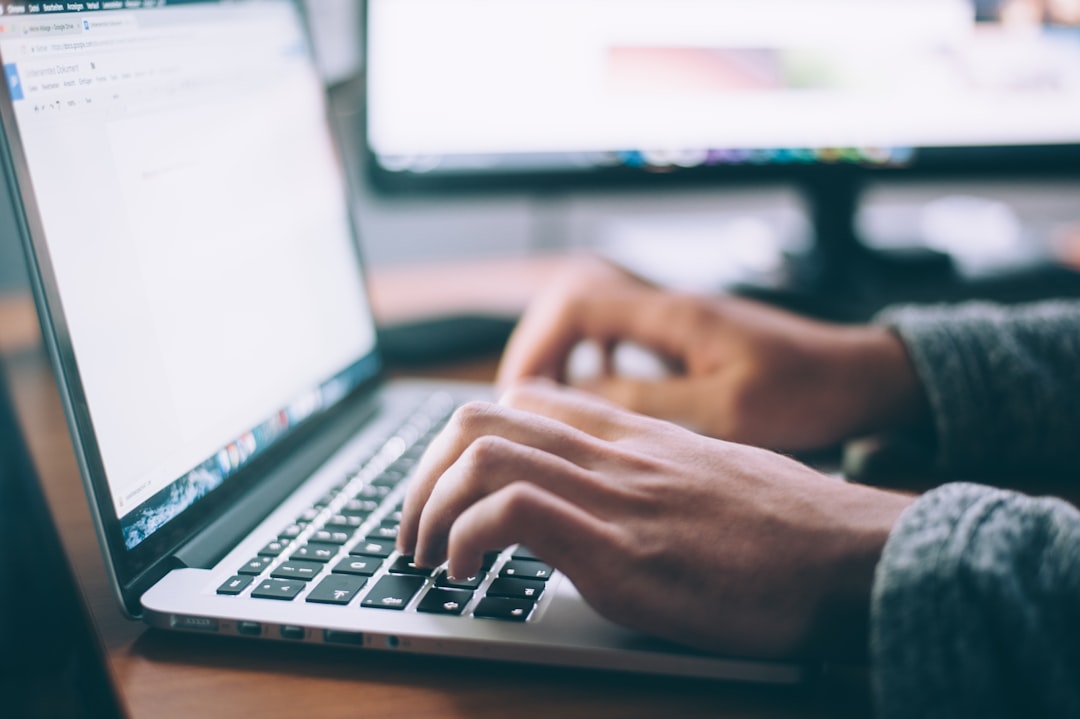
What is WebSocket?
WebSocket is a protocol that is part of the HTML5 standard, allowing bidirectional communication between the client and server. Traditional HTTP communication operates on a request-response basis, whereas WebSocket enables continuous data exchange once the connection is established. This allows the implementation of real-time applications such as chat, online games, and live feed updates.
Features of WebSocket
- Bidirectional Communication: Clients and servers can freely exchange data.
- Real-time: Continuous connections enable real-time data transmission.
- Low Overhead: Efficient due to the lack of HTTP header overhead.
Basic Operation of WebSocket
The operation of WebSocket can be divided into three main stages:
- Handshake: The client requests a WebSocket connection from the server.
- Connection Establishment: The server approves the request, establishing the WebSocket connection.
- Data Frame Exchange: Once established, the client and server continuously exchange data.
WebSocket Implementation Example
We will create a simple chat application using Node.js and Socket.IO.
Server Setup:
const express = require('express');
const http = require('http');
const socketIo = require('socket.io');
const app = express();
const server = http.createServer(app);
const io = socketIo(server);
io.on('connection', (socket) => {
console.log('A user connected');
socket.on('chat message', (msg) => {
io.emit('chat message', msg);
});
socket.on('disconnect', () => {
console.log('User disconnected');
});
});
server.listen(3000, () => {
console.log('Server is running on port 3000');
});
Client Setup:
<!DOCTYPE html>
<html>
<head>
<title>Chat</title>
<script src="/socket.io/socket.io.js"></script>
<script>
document.addEventListener('DOMContentLoaded', () => {
const socket = io();
document.querySelector('form').addEventListener('submit', (e) => {
e.preventDefault();
const input = document.querySelector('#message');
socket.emit('chat message', input.value);
input.value = '';
});
socket.on('chat message', (msg) => {
const li = document.createElement('li');
li.textContent = msg;
document.querySelector('#messages').appendChild(li);
});
});
</script>
</head>
<body>
<ul id="messages"></ul>
<form>
<input id="message" autocomplete="off" /><button>Send</button>
</form>
</body>
</html>
Implementing Secure Real-Time Communication with WSS
In real-time applications, data security is paramount. WebSocket Secure (WSS) enhances WebSocket security by encrypting data, ensuring safe communication. This section covers the basic concepts and implementation methods of WSS.
What is WebSocket Secure (WSS)?
WebSocket Secure uses TLS (Transport Layer Security) to encrypt WebSocket communication, preventing man-in-the-middle attacks and data eavesdropping. It is recommended to use WSS for real-time applications handling sensitive data.
Features of WSS
- Security: Encrypts data using TLS.
- Real-time: Allows real-time bidirectional communication similar to WebSocket.
- Stability: Ensures data integrity and confidentiality.
Basic Operation of WSS
- TLS Handshake: A TLS handshake is performed between the client and server to establish a secure connection.
- WebSocket Handshake: Once the TLS connection is established, a WebSocket handshake is performed.
- Data Frame Exchange: The client and server exchange data over a secure WebSocket connection.
WSS Implementation Example
We will create a simple WSS-based chat application using Node.js.
Server Setup:
const https = require('https');
const fs = require('fs');
const WebSocket = require('ws');
const server = https.createServer({
cert: fs.readFileSync('path/to/cert.pem'),
key: fs.readFileSync('path/to/key.pem')
});
const wss = new WebSocket.Server({ server });
wss.on('connection', (ws) => {
ws.on('message', (message) => {
console.log('received: %s', message);
});
ws.send('something');
});
server.listen(8080, () => {
console.log('Server is running on port 8080');
});
Client Setup:
<!DOCTYPE html>
<html>
<head>
<title>WebSocket Secure Example</title>
</head>
<body>
<script>
const socket = new WebSocket('wss://server.example.com');
socket.addEventListener('open', (event) => {
socket.send('Hello Server!');
});
socket.addEventListener('message', (event) => {
console.log('Message from server ', event.data);
});
</script>
</body>
</html>
Conclusion
WebSocket and WebSocket Secure are highly useful tools for implementing real-time communication. WebSocket enables real-time bidirectional communication with low overhead, while WebSocket Secure adds security. This article aims to help you understand the basic concepts and implementation methods of WebSocket and WSS and apply them to real-time applications.