Many developers who use TypeScript may find its type system complex and difficult to navigate. However, this article aims to provide a fresh perspective on understanding TypeScript’s type system. This approach views TypeScript’s type system as one of a purely functional programming language.
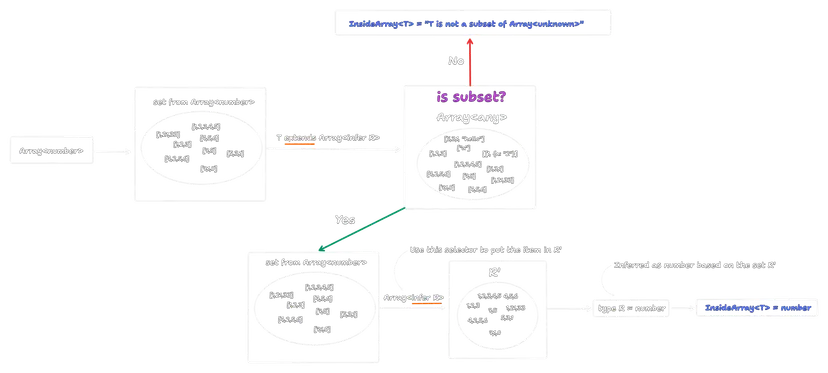
A Fundamental Understanding of TypeScript’s Type System
Let’s reconsider the concept of ‘type’ in TypeScript. Instead of simply seeing a ‘type’ as a way to define the data format for variables or functions, consider it as the **set of all possible values** that can be assigned to that type. For example, the `string` type represents the set of all possible strings, and the `number` type represents the set of all possible numbers. Understanding it this way can make various features of TypeScript much clearer.
Intersection and Union: Viewing Through the Lens of Sets
In TypeScript, intersections (`&`) and unions (`|`) can be better understood through the lens of set theory. An intersection type represents the intersection of two sets, and a union type represents the union of two sets.
For example, `type Foo = { x: number } & { y: number }` is an intersection type, representing the intersection of the two types `{ x: number }` and `{ y: number }`, creating an object **with both x and y as number types**. Conversely, `type Bar = { x: number } | { y: number }` is a union type, creating an object **where either x or y is a number type**.
This perspective helps clarify how intersection and union types behave in different situations.
Type Introspection: Checking Subsets of Sets
Using the `extends` keyword in TypeScript allows you to check if one type is a subset of another, similar to how you might check if one set is contained within another in set theory.
For example, the syntax `type IntrospectFoo = number | null | string extends number ? true : false` checks whether `number | null | string` is a subset of `number`, returning true or false accordingly. This approach allows TypeScript to perform highly effective type checks.
Type Mapping and Transformation: Creating New Sets
TypeScript offers features to iterate over or transform sets, enabling the creation of **new types based on existing ones**. For example, mapped types allow you to create new types based on the keys and values of an object type.
type OnlyBoolsAndNumbers = {
[key: string]: boolean | number;
};
The above example creates an object type **where every key has a value of either boolean or number**. This capability allows for more complex type transformations.
Conclusion: A New Approach to Understanding TypeScript
This new approach to understanding TypeScript can be highly beneficial. By viewing types as **set operations**, we can better grasp and utilize TypeScript’s advanced features. This perspective allows for a deeper understanding than simply memorizing TypeScript’s syntax.
For developers using TypeScript, it is strongly recommended to consider this new perspective on the type system. By doing so, you can prevent more bugs in advance and increase the stability of your code.
Reference: Rob Directory, “A Different Way to Think About TypeScript”